Flask Datta Able - Bootstrap 5 Update
Starter updated to use Bootstrap 5 design, Extended user profiles, CI/CD integration with Render - open-source starter.
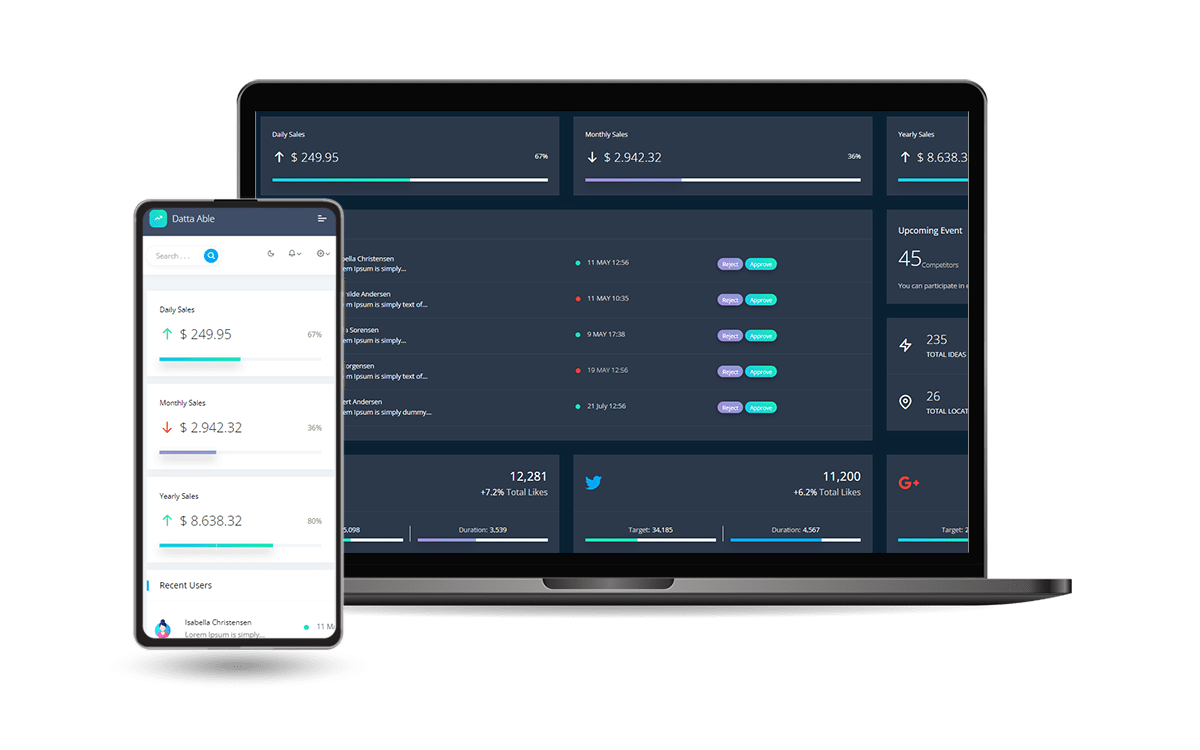
Hello coders! Flask Datta Able has been updated to use Bootstrap 5 styling, Extended Users Profile, One-click deployment for Render, and improved Docker scripts. The product helps developers bootstrap a new dashboard project in minutes and is already configured to be deployed live on Render.
The LIVE Demo deployed on Render showcases the latest version and also contains the support and download links.
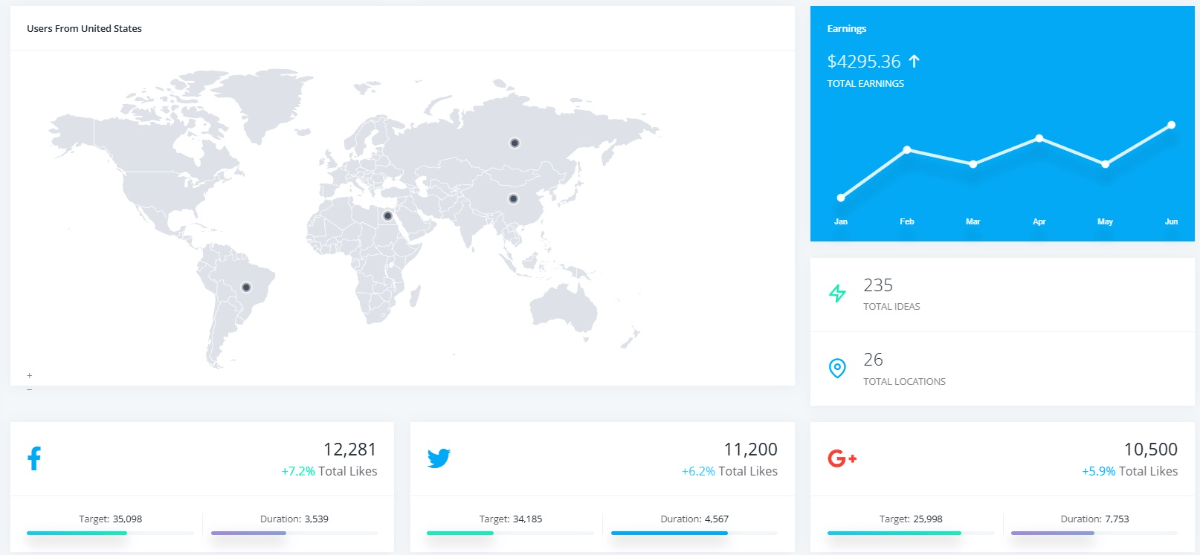
Authenticated users can save their information using this simple page that can be easily extended with more fields and widgets.
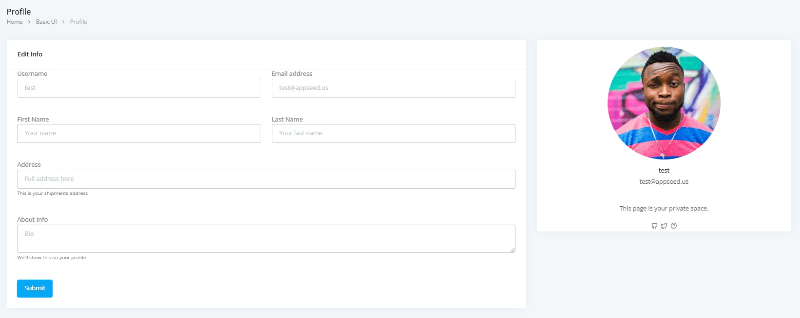
The new Bootstrap 5 layout was polished to ensure an optimal layout on every device. Below are the screens displayed on an iPhone 16.
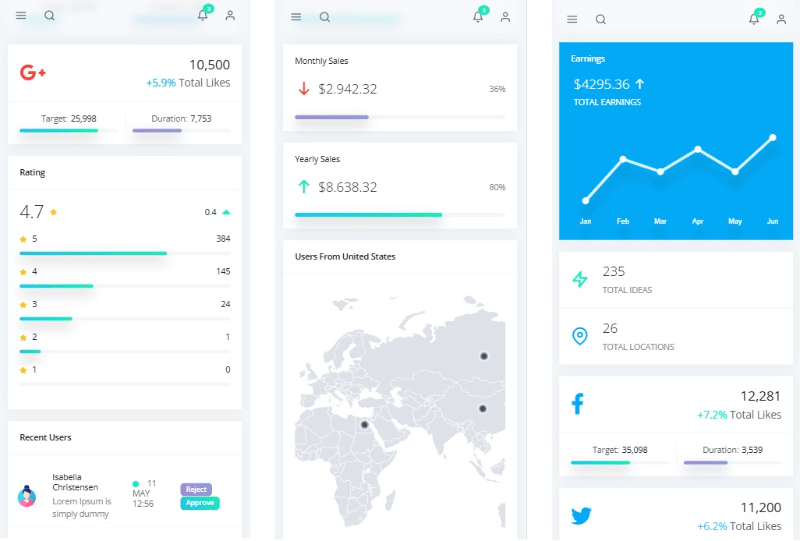
Getting Started with Flask
Flask is a micro web framework for Python that has earned its place as one of the most popular tools for web development. Created by Armin Ronacher in 2010, Flask follows the principle of simplicity without sacrificing flexibility. It's "micro" not because it lacks features, but because it maintains a simple core while being highly extensible.
Why Choose Flask?
- Minimalist but Powerful: Flask provides the essentials without forcing specific structures or dependencies. It gives you the freedom to choose your project's architecture and tools.
- Easy to Learn: With its straightforward syntax and clear documentation, Flask has one of the gentlest learning curves among web frameworks.
- Highly Flexible: Flask can be as simple or as complex as your project requires. It scales from single-file applications to large, feature-rich web services.
- Strong Community: A vast ecosystem of extensions and a supportive community make it easy to find solutions and add functionality.
Here's a practical example that demonstrates Flask's core concepts:
from flask import Flask, request, render_template
# Create Flask application instance
app = Flask(__name__)
# Define routes using decorators
@app.route('/')
def home():
return 'Welcome to Flask!'
# Route with dynamic parameter
@app.route('/user/<username>')
def show_user(username):
return f'Hello, {username}!'
# Route handling different HTTP methods
@app.route('/submit', methods=['GET', 'POST'])
def submit():
if request.method == 'POST':
# Handle form submission
data = request.form['data']
return f'Received: {data}'
# Show form for GET requests
return '''
<form method="post">
<input type="text" name="data">
<input type="submit" value="Submit">
</form>
'''
# Template example
@app.route('/greet/<name>')
def greet(name):
return render_template('greet.html', name=name)
if __name__ == '__main__':
# Run the application in debug mode
app.run(debug=True)
The above project can be started and visited in the browser after installing the Flask library and starting the project:
pip install flask
python app.py
At this point, our minimal Flask project runs at http://localhost:5000.
Flask's simplicity makes it an excellent choice for both beginners and experienced developers. Whether you're building a simple website or a complex web service, Flask provides the tools you need while staying out of your way.
Thanks for reading! For more resources and support please access:
- More Flask Dashboards - starters actively supported by App-Generator Platform
- Get Support via email and Discord (for registered users)